Introduction to COSMAC 1802 OR & AND Statements
COSMAC 1802 OR & AND instructions allow us to manipulate data. These instructions work on all 8 bits of a word at a time. These instructions work just like standard bit level logic gates work. They just perform the operation bit by bit on all 8 bits.
In this section, we’ll take a look at the various OR & AND instructions available in the 1802. Additionally, we’ll discuss ways that we can use them in our code.
I’ll be using the A18 Assembler in this post.
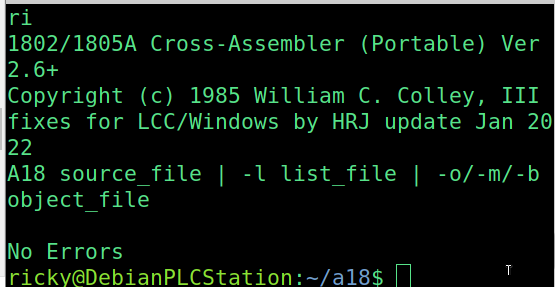
Basically, both instructions will look at two words of data. Let’s consider only bit zero for a second. If we look just at bit 0, and in both of the words, bit 0 is high, then the AND statement is true. Therefore, you will have a 1 in the destination. On the other hand, the OR statement works a bit differently. only one of the two bits need to be high to get a 1 in the destination. There is also a XOR instruction. With the XOR instruction, one or the other must be high, but not both, to get a 1 in the destination.
Let’s take a look at the result of 2 words. Look at these one column at a time.
OR XOR AND
1100 0101 1100 0101 1100 0101 (C5 Hex)
1001 0111 1001 0111 1001 0111 (97 Hex)
_________ _________ _________
1101 0111(D7H) 0101 0010(52H) 1000 0101(85H) <-- Results
OR Type Statements (COSMAC 1802 OR & AND Instructions)
We have two types of OR statements in the COSMAC: OR, and ORI. OR works with a memory location. X points to the scratchpad register that contains the address of the data. We OR this value with what is in the accumulator. The result goes back to the accumulator.
On the other hand, we have an ORI instruction. This instruction will OR an immediate value with the accumulator. The result goes back to the accumulator.
At this point, let’s take a look at some logic to see how these instructions would operate.
OR Instruction:
Remember, when we OR words together bit for bit, we only need one bit or both bits to be true in any particular bit location. Let’s say our COSMAC can start 8 motors. One memory location might represent an Operator request to energize any motor. Another memory cell would be the automatic requests. If either the manual or automatic bit goes true at any bit position, then we write a 1 to the same bit position of the destination. This bit position in the destination will go high. The motor will start.
BREAK: EQU 2765H
VALUE1: EQU 8200H
VALUE2: EQU 8201H
VALUE3: EQU 8202H
RA EQU 10
RB EQU 11
RC EQU 12
ORG 8000H
INIT: LOAD RA,VALUE1
LOAD RB,VALUE2
LDI 0C5H
STR RA
START: LDI 97H
SEX RA
OR
STR RB
END: LBR BREAK
END
Test your work! Memory cell 8201H should contain the value of D7H.
ORI Instruction
ORI will OR the accumulator with an immediate value. We don’t need a whole lot of logic to make this one happen. Let’s take a look at how we would do the same thing as above with the ORI instruction.
BREAK: EQU 2765H
VALUE1: EQU 8200H
RA EQU 10
ORG 8000H
INIT: LOAD RA, VALUE1
START: LDI 0C5H
ORI 97H
STR RA
END: LBR BREAK
END
Take a look at memory location 8200 at this point. The value should be D7H.
XOR Type Statements (Exclusive OR)
At this point, we’ll take a look at the XOR instruction. We use the XOR to reverse bits. In other words, we just turn on bit positions in a mask that we want to reverse in the source.
Effectively, we can use this to compare two numbers as well. If we XOR two numbers that are the same, then the result is zero. We can use this before on a BZ (Branch when D Zero) instruction. Let’s say we are executing a loop, and the loop is running up toward the value of 100. If we save the accumulator to memory, then XOR it with 100, the accumulator will be zero when our loop reaches 100 counts (hex).
Let’s take a look at a couple of examples.
XOR (Exclusive OR) Instruction
The XOR statement will XOR a memory location with the accumulator. The result goes back to the accumulator. The memory location of the source value is in a register that X designates.
BREAK: EQU 2765H
VALUE1: EQU 8200H
VALUE2: EQU 8201H
VALUE3: EQU 8202H
RA EQU 10
RB EQU 11
RC EQU 12
ORG 8000H
INIT: LOAD RA,VALUE1
LOAD RB,VALUE2
LDI 0C5H
STR RA
START: LDI 97H
SEX RA
XOR
STR RB
END: LBR BREAK
END
Take a look at address 8201. Your result should be 52H.
XRI (XOR Immediate) Instruction
The XRI will XOR the accumulator with an immediate value. we specify the immediate value after the XRI instruction. Let’s take a look at this, and see if we get the results we expect.
BREAK: EQU 2765H
VALUE1: EQU 8200H
RA EQU 10
ORG 8000H
INIT: LOAD RA, VALUE1
START: LDI 0C5H
XRI 97H
STR RA
END: LBR BREAK
END
Check memory location 8200H. If it worked, then you should have the value of 52H.
AND Type Statements( COSMAC 1802 OR & AND Instructions)
We use and object when we want to require the bit position of 2 words to be high in order to write a 1 to the destination. We can use the AND statements as permissives. For example, I turn on bit 7 in the source. Bit 7 must also be turned on in the compare word to get a 1 on the destination. Both bits of the same position must be high for the destination bit to be high.
AND Instruction
We use the AND statement to AND a word of memory with the accumulator. The location of the memory data is in a register that X designates. Let’s take a look at some code.
BREAK: EQU 2765H
VALUE1: EQU 8200H
VALUE2: EQU 8201H
VALUE3: EQU 8202H
RA EQU 10
RB EQU 11
RC EQU 12
ORG 8000H
INIT: LOAD RA,VALUE1
LOAD RB,VALUE2
LDI 0C5H
STR RA
START: LDI 97H
SEX RA
AND
STR RB
END: LBR BREAK
END
Take a look at address 8201H. The result should be 85
ANI Instruction
At this point, we’ll look at the ANI statement. This will simply AND the accumulator with an immediate value. We place the immediate value right after the ANI Instruction. As I said before, the result goes back to the accumulator.
BREAK: EQU 2765H
VALUE1: EQU 8200H
RA EQU 10
ORG 8000H
INIT: LOAD RA, VALUE1
START: LDI 0C5H
ANI 97H
STR RA
END: LBR BREAK
END
At this point, take a look at address 8200. Obviously, the result should be 85 as before.
For more information, visit the COSMAC Category Page!
— Ricky Bryce