Introduction to the Kim-1 (UNO) GETKEY Routine
The Kim-1 (UNO) GETKEY Routine allows us to accept user input. When the user presses a key, we can take action on that key. The GETKEY routine resides at $1F6A on the Kim Uno. In this post, we’ll write a simple program that will get the last key pressed, then display this key to the display. This will prove that our keys are working properly.
It’s important to realize that when we don’t press any keys at all, the GETKEY routine returns “FF” on the Kim UNO. When $1F6A returns a value, it stores this value to the accumulator. In other words, if there is any data you want to save from the accumulator, use the STA command to store it somewhere in memory before running the routine. Afterwards, you can use the LDA command to retrieve the previous value.
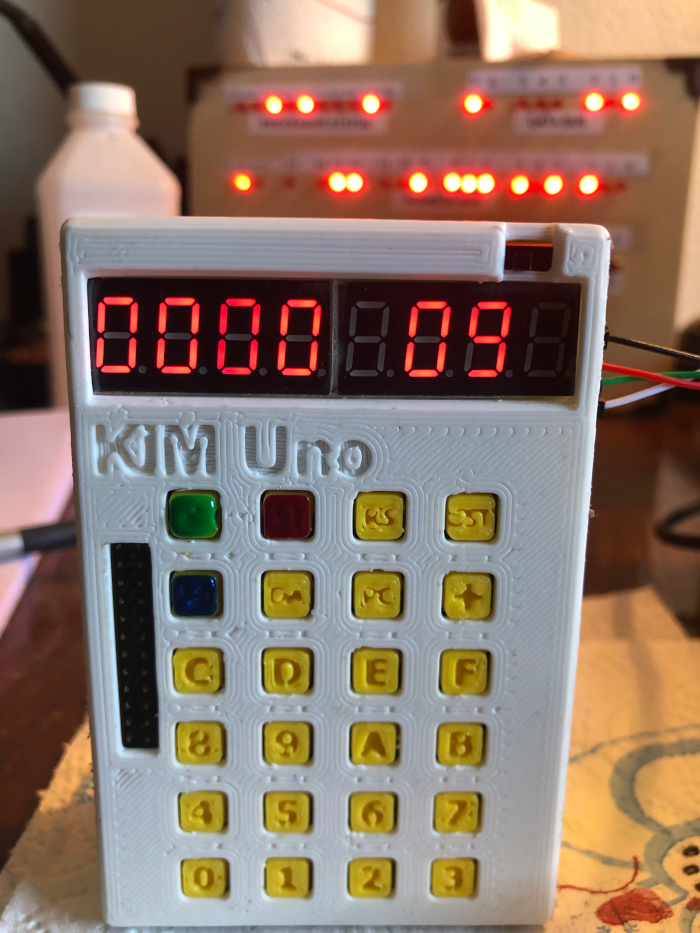
Write the Program
To begin with, I’ll explain the code in assembly. There is a good assembler at this link. Afterwards, I’ll do a hex dump if you wish to try the program on your own. Our program will start at memory cell $0200. In assembly, we’ll start with this line:
.ORG $0200
Initialize the display for the Kim-1 (UNO) GETKEY Routine
At this point, we’ll load all zeros to the display. This will keep us from getting confused later on. Cells $FB, $FA, and $F9 will show up on your Kim-1’s display once we run the routine at $1F1F. Therefore, we’ll initialize these values before we begin.
LDA #$00
STA $F9
STA $FA
STA $FB
Display the Values
At this point, we’ll want to keep updating the display, showing the values of these three memory locations. We’ll place a label in our project that we can jump back to. After that, we’ll execute the subroutine at $1F1F. This will refresh our display every time we jump back to this label.
DISPLAY:
JSR $1F1F
Run the Kim-1 (UNO) GETKEY Routine
At this point, we’ll run the GETKEY subroutine. This will execute the subroutine at $1F6A. It will store this result to the accumulator.
JSR $1F6A
Test the Accumulator
At last, we are ready to test the accumulator to see if we have a valid key. If they key is invalid (FF), then we don’t want to display this, so we’ll just jump back to the DISPLAY label. However, if it is any other value, we will move the result to $F9 before we jump back to the DISPLAY label.
When we use the CMP statement, the processor will set the zero flag if the result is FF. The BEQ in the next line looks for this zero flag. If it’s high, then we know the result was FF. Therefore, the BEQ will jump back to the DISPLAY label.
However, if the result is any other value, we will move that value to $F9. Once we jump back to the display label, our SCANDS routine at $1F1F picks up this value. It will be stored to the right-most digits of your KIM.
CMP #$FF
BEQ DISPLAY
STA $F9
JMP DISPLAY
Full Code
Here is a listing of the full code in assembly. I simply plug this code into the assembler at masswerk. After that, I shut off the addresses for the object code window. I’ll then paste that into kwrite, and delete the carriage returns. Finally, I replace the spaces with a period, then copy the code and paste it into the minicom terminal so it will start loading at $0200. Be sure to set up your displays, or your code will fail. I have the character delay, and new line delay set at 10ms.
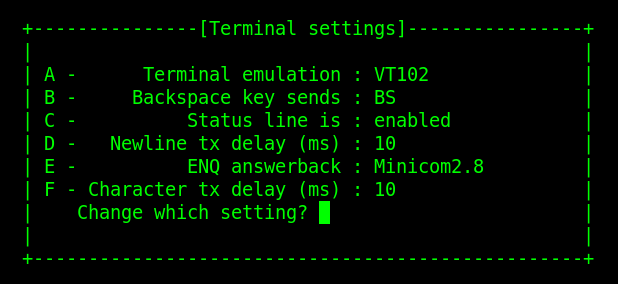
Here is the full code:
.ORG $0200
LDA #$00
STA $F9
STA $FA
STA $FB
DISPLAY:
JSR $1F1F
JSR $1F6A
CMP #$FF
BEQ DISPLAY
STA $F9
JMP DISPLAY
Then here is the hex dump:
A9.00.85.F9.85.FA.85.FB.20.1F.1F.20.6A.1F.C9.FF.F0.F6.85.F9.4C.08.02.
Summary of Kim-1 (UNO) GETKEY Routine
Use the GETKEY routine any time by running JSR $1F6A. This result goes to the accumulator. After that, you can use the CMP instruction to compare it against any value. After the CMP instruction, you can use the BEQ instruction to branch somewhere else in your program if the key equals a specific value. Otherwise, you can use the BNE instruction if you want to branch when the value does not equal the value you specify as the operand the CMP statement.
For more information, check out the vintage computers category page!
— Ricky Bryce