Introduction to COSMAC 1802 Basic Branching
COSMAC 1802 Basic Branching instructions allow you to execute different parts of code based on certain conditions. These conditions might include inputs that are high, or results of an operation that return a zero value, negative value, etc. In this section, we’ll cover the branching instructions, and how to use each one.
BR, LBR, LBZ, BZ, BNZ Instructions
We don’t need to go through every one of these, but I’ll explain how they work. The BR is a short branch instruction. That is to say, it will branch to a program location that is on the same memory page. In other words, we only need to specify the last byte of an address to branch to.
The LBR is a long branch instruction. This instruction will branch anywhere in memory. When we perform a long branch, we specify both the low and high bytes of the address we need to branch to.
BZ will execute a short branch when the accumulator is zero. Use LBZ if you need a long branch when the accumulator is zero. In the example below, we’ll run up the accumulator to the value of 09, using the low byte of RA as a storage location. We will use the XRI (Exclusive Or Immediate) instruction to know when we reach 09H. When we XOR a value with the accumulator, the result is zero if the numbers are the same. In this case, when the accumulator reaches 09H, and we XOR the accumulator with 09H, the result is zero. At this point we can branch to the stop label.
Our BNZ instruction will branch when the accumulator is not equal to zero. Basically, this is the opposite of BNZ.
Everyday Branch Logic
Let’s take a look at some logic. These are branch instructions that you will probably use in every program. This logic will loop a total of 10 times (0 to 9). After the accumulator reaches 9, the result of our “XRI 09H” will result in a value of zero in the accumulator (D Regsiter). That this point, we branch to the STOP label. In my unit, I have some ROM that will execute a break routine to load the registers into memory before the processor stops. The break routine is on a different memory page. In other words, we need a long branch (LBR) to execute this routine.
BREAK: EQU 2756H
ORG 8000H
RA EQU 10
LDI 00H
PLO RA
PHI RA
START: GLO RA
ADI 01H
PLO RA
XRI 09H
BNZ START
BR STOP
STOP: LBR BREAK
END
Test your work! Register RA (Register 10) should contain the value of 09H once your program finishes.
EF and Q Branches
At this point, we’ll take a look at branches that operate of the value of your EF and Q bits. You have four EF bits that directly connect to the processor (5v = 0, 0v = 1). You also have a Q output on the processor. In this case, my Q output connects to an LED. I have two buttons on my unit that connect to EF1 and EF2.
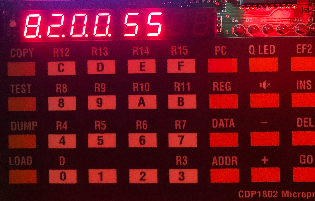
In this example, we’ll turn on the LED with the SEQ (Set Q) instruction when we press EF1. Likewise, when we press EF1 again, Q shuts off. The cycle repeats infinitely.
B1 – B4 will branch when we press the button. BN1 – BN4 branch when we are not pressing the button. Likewise, BQ branches when Q is on, and BNQ branches when Q is off.
Let’s take a look at the logic:
BREAK: EQU 2756H
ORG 8000H
RA EQU 10
LDI 00H
PLO RA
PHI RA
START: BN1 CLEARFLAG
GLO RA
BNZ NOACTION
B1 EVENTFLAG
NOACTION: BR START
EVENTFLAG: BQ REQ
SETQ: LDI 01H
PLO RA
SEQ
BR START
REQ: LDI 01H
PLO RA
REQ
BR START
CLEARFLAG: LDI 00H
PLO RA
BR START
END
Summary of COSMAC 1802 Basic Branching
In short, branches allow you to jump to different areas of memory based on certain conditions. You can consult the COSMAC 1802 user manual for more types of branches. Here, I’ve just listed a few as an example. Once you learn how the above branches work, the others will mostly be self-explanatory.
For more information, visit the COSMAC Category Page!
— Ricky Bryce