Introduction to Serial Write on the Southern Cross
This post is for absolute beginners who which to perform a Serial Write on the Southern Cross Z80 Computer. Craig Jones develops and maintains the Southern Cross Z80 Computer. In this section, I’ll be using the ACIA Serial Monitor, and the SCNEO ROM.
For this post, I’ll just be using a standard text editor. I’m also using the AZ80 assembler. We’ll simply write “Hello World!” through the ACIA module back to your terminal.
Set Originating Location and Initialize Registers
In each program, we need to use the ORG statement. The ORG statement will tell the assembler where your code begins. This is important for jumps, and system calls. Otherwise, the assembler would jump to addresses that we do not expect. Additionally, I always like to initialize the registers that I use in code. This sets the registers to a known value. This is not always necessary since we will usually redefine these values in logic anyway.
Be sure that instructions have a tab or a few spaces before them. Otherwise, our assembler may interpret these as labels. Hexadecimal values will have the letter “H” after them. If a hexadecimal value starts with a letter, such as FF, then you will also need a 0 before the value. ie. 0FFH will be the hex value FF.
Later on, we will use the C register for function codes on system calls. The Southern Cross SCNEO monitor has system calls that perform routine tasks for us. We’ll be using those system calls in this code. Some system calls require us to pass additional data via the HL register. The “A” register is the accumulator. That is our main working register that we use in code.
ORG 2000H ; PROGRAM STARTS AT 2000H
; ** BEGIN INITIALIZE **
INIT: ; INITIALIZE REGISTERS AND MEMORY
LD A,0 ; ZERO ACCUMULATOR
LD C,0 ; ZERO C REGISTER FOR SYSTEM FUNCTIONS
LD HL,00H ; ZERO HL FOR SYSTEM FUNCTIONS
; ** END INITIALIZE ***
Write the Main Routine for our Serial Write on the Southern Cross
At this point, we are ready to write the main routine. We need to set up some registers before performing our system call. First, we have a label, BEGIN: Later on, if we wish to loop the program, we can always jump back to the beginning of our code.
Next, we LD HL,MYMSG. In the next section of this post, we will define what the message is. This will simply load the HL register with the address that our message begins at.
Next, we load C with the decimal value of 34. This is a function call in the system that will write the message to the serial port. When the system sees a 00H, then it knows to terminate the message. If you use the Southern Cross “INCLUDE” file, then you could simply use LD C,SNDMSG. The INCLUDE file defines the value of SNDMSG as 34. Here, I’ve just shown that code as a comment. Anything after a simicolon “;” on a line is a comment for the programmer, or anyone viewing the code. The assembler ignores comments (for the most part). Obviously, they will still appear on a list file.
Lastly, we call the system with RST 30H. Although you can use a call to 30H, the RST instruction is more efficient and uses fewer processor cycles. There are only specific addresses the RST instruction supports.
Finally, we stop the processor with our HALT instruction.
; ** BEGIN MAIN ROUTINE **
BEGIN:
LD HL,MYMSG
LD C,34 ;LD C,SNDMSG
RST 30H
DONE:
HALT
; ** END MAIN ROUTINE
Composing the Message to Write
Finally, we are ready to write the message. Remember that previously we loaded HL will the memory location of MYMSG. Now, it’s time to define that message. First, we have a label, MYMSG:. After that, we will define a starting byte with the DB instruction. You will notice that “Hello World!” is in quotes. This tells the assembler to convert this text to ASCII. After that, we have a 0DH, which is a carriage return. Then we have 0AH, which is a line feed. We terminate the string with 00H.
; ** BEGIN DATA TABLES (ARRAYS) **
MYMSG: ; Message that we display
DB "Hello World!", 0DH, 0AH, 00H
; ** END DATA TABLES (ARRAYS) **
Assembling your Project
We’ll save the project named “SimpleSerialWrite.asm”. At this time, we will run the AZ80 Assembler. I’m using Linux, so I simply enter the directory that my assembler and type ./az80 SimpleSerialWrite.asm -l SimpleSerialWrite.lst -o SimpleSerialWrite.hex
The AZ80 assembler will build your hex code that you will now send to the Southern Cross. In your terminal, reset the Southern Cross, and hit the Fn key twice. type I, then send your file. After that, type G 2000 to run your program starting at memory cell 2000H
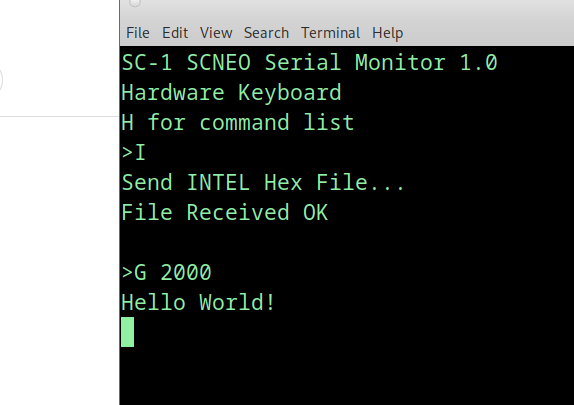
The final hex code:
:102000003E000E00210000210E200E22F7764865CA
:0D2010006C6C6F20576F726C64210D0A001C
:00201D01C2
For more information, visit the Vintage Computers Category Page!
— Ricky Bryce