Introduction to COSMAC UART Communication
I’ll be using the CDP1802 Microprocessor kit to demonstrate COSMAC UART Communication. This setup uses the INS8250 chip for communication. The monitor initializes the IC. In this section, we’ll be sending data out of the serial port. UART is short for Universal Asynchronous Receive and Transmit.
We need to be concerned with two addresses for this feature. $7300 will be the buffer to which we store our data for transmit. $7305 is for status. Basically, we dump a byte ASCII data into the buffer, and then wait for bit #5 to go high. To check for this bit, we simply load the accumulator with the status data, and AND this data with 20H. If this bit did not go high, we just continue in the loop until the buffer is ready to receive another character.
This feature is good for communication as well as troubleshooting. When the program is running, we can send out bytes to indicate the status of various registers.
Logic for COSMAC UART Communication
Header
At this point, we’ll take a look at some logic to simply write the word “HELLO!” out of the serial port. First, we’ll need to initialize some registers. This will declare the memory location for the serial port status, buffer, loop, and break. Additionally, we need to set up the register declarations if your assembler does not automatically do this.
STATUS: EQU 7305H
BUFFER: EQU 7300H
LOOP: EQU 8200H
BREAK: EQU 2756H
R0 EQU 0
R1 EQU 1
R2 EQU 2
R3 EQU 3
R4 EQU 4
R5 EQU 5
R6 EQU 6
R7 EQU 7
R8 EQU 8
R9 EQU 9
RA EQU 10
RB EQU 11
RC EQU 12
RD EQU 13
RE EQU 14
RF EQU 15
Load Registers
Next, we’ll load up our registers. Since I’m using the CDP1802 Microcontroller kit, my address will start at $8000H. In the TEXT database, we will set up the text we wish to send to the terminal. In this case, it will be the word “HELLO!”. Keep in mind this is a total of six characters. We need to store the number of characters to R6 because this is how many times we need to loop through our logic. Once for each character.
org 8000H
TEXT: DB 'H', 'E', 'L', 'L', 'O', '!'
LOAD R4, STATUS
LOAD R5, BUFFER
LOAD R6, LOOP
LOAD R7, TEXT
LDI 06H ;SET UP NUMBER OF LOOP CYCLES
STR R6
Status Loop
At this time, we get into the actual logic that will deal with handling the UART. We will need to check the status of the serial connection. We are waiting for bit #6 to go high. Once this goes high, we are ready to send the next characters. We’ll just remain in this loop until we get our OK flag.
START: LDN R4 ;CHECK FOR RESPONSE OK
ANI 20H
BZ START
Writing Data to Buffer
At last we are ready to decrease our loop counter, and send each character into the buffer.
LDA R7
STR R5
SEX R6
LDI 01H
SD
STR R6
LDN R6
BZ LONGBREAK
BR START
LONGBREAK LBR BREAK
END
We will Load from R7 and advance the pointer (LDA). That way, we are set up to get the next character the next time we execute the LDA. We will store this value to R5, which is our buffer. Remember, the INS8250 IC will send the character in the buffer to our terminal.
At this point, we’ll decrement the loop counter. We the X designator to point to R6. After that, we load the value of 1 into our accumulator (D Register). When we execute the SD instruction, the COSMAC will subtract the accumulator from R6, then store the result back to R6. This effectively decreases our loop counter by 1.
At this point, I have a habit of reloading the value into R6 just to be sure nothing has changed. We will check this accumulator to see if it is zero. If so, we branch to LONGBREAK which effectively stops the processor.
Obviously, if the value in R6 is not yet zero, then we go back to the start of the logic to print the next character.
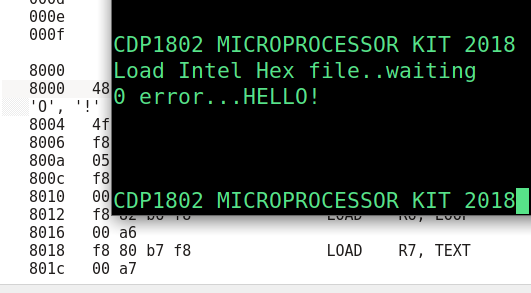
Run your program, and you should see the word HELLO!
Intel Hex File for COSMAC UART Communication
Here is a HEX dump of this simple logic. You can use this if you have the ability to load Intel Hex files into your COSMAC unit.
:2080000048454C4C4F21F873B4F805A4F873B5F800A5F882B6F800A6F880B7F800A7F806B4
:158020005604FA2032214755E6F801F5560632323021C02756C6
:008035014A
For more information, please visit the COSMAC category page!
— Ricky Bryce