Introduction to Assembly Registers and Compatibility Modes
Before we begin to program, we need to understand Assembly Registers and Compatibility Modes. In this case, we’ll discuss Assembly Language with the x86 / x64 processors. Assembly is a low level language that allows you to write machine code without the need to memorize instruction codes (OPCODES). Instead, we just use mnemonics.
It’s important to realize there is a difference between Registers, and Memory locations. Registers reside on board the processor itself. You have a limited number of registers. Modern machines have a lot of memory. In our assembly programs, memory is usually not an issue unless we work with large arrays. However, working with memory locations is much slower than working with registers.
Think of your tool box when working on your vehicle. When you get the tools out, and they are in front of you, they are very easy and fast to access. The same is true for registers. If we need to go to the toolbox, though, it takes longer to access the tool we need. This is similar to accessing memory in the machine. It’s important to realize that we do not always use these registers for their intended purpose. For example, ESI and EDI can both be used to pass a first and second parameter into a function.
Registers for x86 Machines
For x86 machines, we have 8 main registers that we’ll be working with. These are 32 bit registers.
- EAX — (ACCUMULATOR) This is our primary register.
- ECX — (COUNTER) Here, we usually store the value of loop counters
- EDX — (DATA) Temporary Data Storage
- EBX — (BASE) This is usually our base address for indirect or indexed addressing
- ESP — (STACK POINTER) This tells the processor the location of the current stack value.
- EBP — (STACK BASE POINTER) Base address for the stack. We usually use this in function calls, so we know where parameters are stored.
- ESI — (SOURCE) For String instructions, this will be the source Index. We also use this as the first parameter we pass to functions.
- EDI — (DESTINATION) For String instructions, this is the destination index. We also use this as the second parameter we pass to functions.
Registers for x64 Machines
x64 machines have the same registers as the 32 bit machines with a couple exceptions. First of all, the registers are 64 bit instead of 32 bit. Secondly, all of these registers begin with the letter R instead of the letter E. For example, we name a 64 bit accumulator as “RAX”. These are backward compatible. You can still use these registers in 16 bit mode by using the 16 bit mnemonic, for example EAX.
We also have additional registers for x64 machines, which are R8 to R15. R8 to R11 are “scratchpad registers”. R12 to R15 are reserved.
Other Assembly Registers and Compatibility Modes
We can also specify the registers to be compatible with 16 bit and 8 bit modes. For 16 bit register mode, simply drop the first letter of the mnemonic all together. ie: AX, CX, DX, BX, SP, BP, SI, DI.
On the other hand, for 8 bit addressing, we simply break the relevant registers down into a low and high byte. However, the Stack Pointer, Stack Pointer Base, Source Index, and Destination Index can only be a single value anyway. The result is that we end up with a few more registers, because we are breaking the first four registers into a high and low byte. This gives us the following registers in 8 bit mode: AH, AL, CH, CL, DH, DL, BH, BL…. Then we have the last four registers: SPL, BPL, SIL, and DIL.
Summary of Assembly Registers and Compatibility Modes
In short, registers are faster than memory. Organize your program in a way to use the registers as much as you can, and reduce the number of times that you need to access memory locations. Please comment if you find any discrepancies in this document, and I will correct them as soon as I can. In another post, we’ll get into the different addressing modes, and how to use these in a program, but here is a screen shot of a simple program that I entered from Jonathan Bartlett’s programming examples. As you can see, we use registers extensively in programs.
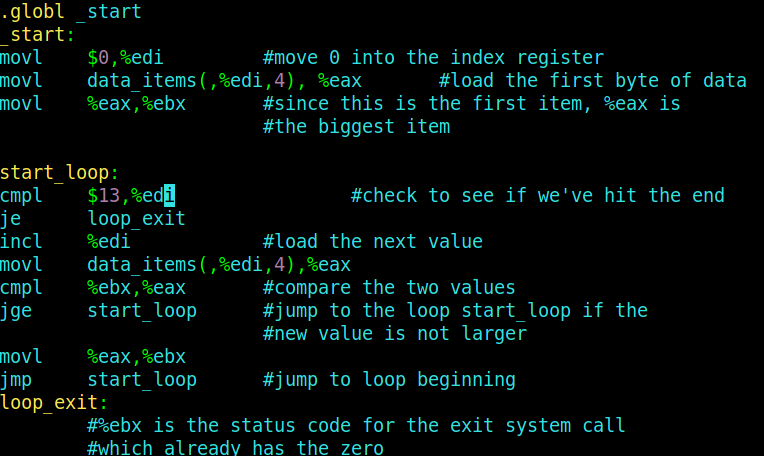
For more information, visit the information technology page!
— Ricky Bryce