Creating Hello World in Modern Assembly
To create Hello World in Modern Assembly (x86 / x64) we’ll need to have an assembler installed. In this case, I’m using the GNU Assembler (GAS). I’ll set this up in Debian Linux. This assembler is in the “build-essential” package. We’ll walk through the creation of this project step by step. After that, we’ll assemble, and load the file (through our linker). Then we’ll test the project.
The purpose of this project is to simply send the text “Hello World!” to the terminal. This walks you through getting a very simple project up and running. After that, you can research other assembly instructions, and tweak the program. A Hello World project is always a great starting point for learning assembly.
Add Data and Text Areas
This assembly program (as well as many others) contain two main sections. The data area is where we store data that we need to sue in the project. The Text area is where we write our program. In other words, the text area contains instructions.
At this time, we’ll start off with some comments. Let’s enter these comments, the data area, and we’ll start the text area of our program. It’s important to realize, that the label “mystring:” will represent the memory location of the start of our string. We’ll declare this data as ASCII, and at the end of “Hello World!”, you notice we have a \n. This will start a new line on the terminal.
# PURPOSE: The purpose of this program is to simply demonstrate
# How to send a string output to the terminal.
# INPUT: NONE
# OUTPUT: Hello World!
#
# NOTE: After the program runs, you can view the status
# by typing echo #?
.section .data
mystring:
.ascii "Hello World!\n"
.section .text
Populate the Text Area for Hello World in Modern Assembly
In this area, we will enter the program. Let’s type in the rest of our program, then we’ll walk through this step by step.
.globl _start
_start:
mov $1, %eax # System Call to Write
mov $1, %edi # Write to screen (stdout)
# Sends output to Terminal
mov $mystring, %esi # Address of String to send
mov $13, %edx # String Length (bytes)
syscall
#Exit
mov $0, %rdi
mov $60, %rax
syscall
First, we are declaring the start label as GLOBAL. This allows the linker to see where our program starts.
After that, we need to set up a few registers. We are setting up these registers to tell the system what to do with the data. The Accumulator (eax) will contain the value of 1. This tells the system that we want to perform a write. Check out this chart for a list of system calls. After that, we need to tell the system what to write to. By placing the value of 1 into the edi register, we are instructing the system to send the output to our terminal (stdout).
Additionally, the system needs to know where in memory the string resides. We move the starting address of the string into the esi register. Obviously, the system also needs to know how much data to write. The length will be 13 bytes (13 characters) total. We place this value into our data register (edx).
At last we are ready to call the system to take action with the syscall command.
After that, we will cleanly exit the program. In this case, I’m moving 0x3c into the accumulator. Then we are ready to take action with the syscall command.
We’ll save our work as “hello.s”.
Assemble and Link your Code
I’m cheating a little bit by making a script. As you can see, this script makes the common task of assembling and linking files a little bit easier. In order to write the script, I’m just using VI. You can use your favorite editor.
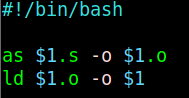
Now press :wq to write and quit. Next, we need to make this script executable: chmod 766 assemble
In this example, I have my script in the same directory as the file I created. I’ll just type “./assemble hello”. The script will add the suffixes we need automatically.
If you didn’t get any errors, let’s try running the program:
./hello
Your result should appear on your terminal screen.

If you have trouble, look at every character in your hello.s file. Chances are, you have a mistake in your code. Here is my full listing:
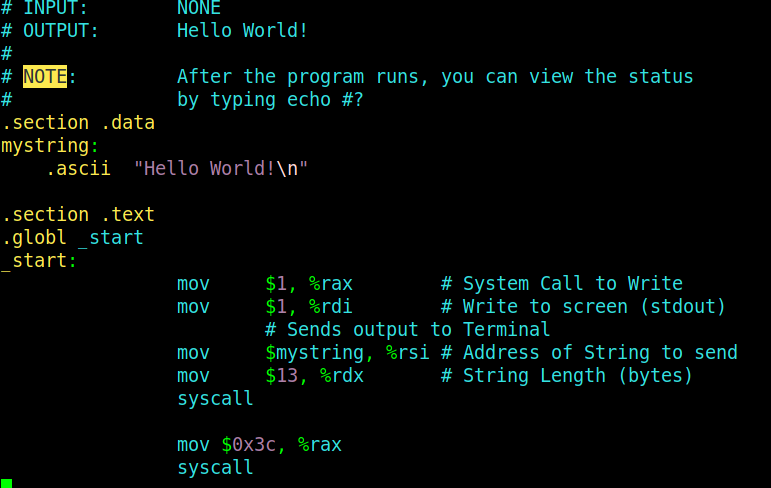
For more information, visit the information technology category! As always, if you see any errors in this post, feel free to comment below, so I can get them corrected.
— Ricky Bryce