Introduction to Assembly Programming Tips
In this section, I’m compiling a list of Assembly Programming Tips. Mainly, this is for manipulating data in assembly language. Most of these methods will work with any processor. It’s important to realize, though, that you might have special instructions in some processors that handle these operations more efficiently. A growing community is working with vintage processors such as the Z80, 8088, 6502, and COSMAC 1802. If you are working with one of these processors, and do not have an instruction available for certain tasks, then these tips might help you. In time, I will be adding to this list as I come across them. Feel free to leave more helpful tips in the comments section at the bottom of this post.
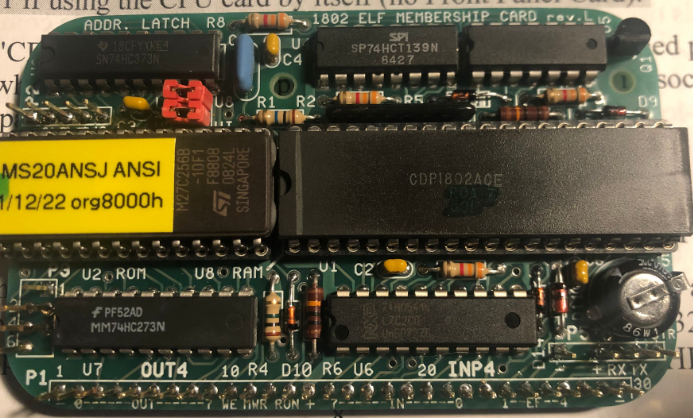
Left Shift or Right Shift
To shift all of the bits to the left within a word, simply add the value to itself. For example: 0111 in binary would be 7 in decimal. Likewise 1110 in binary would be 14 in decimal.
For a right shift, simply cut the value in half, disregarding the remainder. Your right-most value is lost.
This actually works with any numbering system. Simply multiply by the base of the numbering system to move the digits left. Divide by the base of the numbering system to move the digits right. For example: If we take 10 in decimal , and multiply this by the base (which is 10), then we end up with the value of 100.
Set or Reset a Single Bit within a Word
Sometimes, we need to work with individual binary bits. This especially applies to discrete devices, such as switches, or LED’s.
To SET a bit within a word, simply OR the register with a value that has the proper bit set. For example, if we want to energize bit 3 in this pattern: 0111, we simply OR it with 8d (1000). We end up with 1111, which is 15d.
To RESET a bit in a word, we simply AND the register with another word that ONLY has the bit off that we want to shut off. Let’s say the accumulator contains 0000 0111b. If we want to shut bit 1 off, we would AND this value with 1111 1101. We end up with 0000 0101.
Toggle or Reverse all Bits
To TOGGLE a bit within a word, we would use XOR. Again, let’s say that our accumulator contains 0000 1001. We want to toggle bit 3, so we would XOR the accumulator with 0000 1000. The result would be 0000 0001.
To REVERSE ALL BITS within a word, you can XOR a word with FF (for 8 bit words), or -1d. Occasionally, it’s necessary to reverse all bits if your output is active low. For example, if you are using a transistor to energize an LED, you might need to send a LOW signal to the transistor to turn the LED on. Likewise, a HIGH signal might turn it off.
Zero a Register
It’s easy enough to zero a register by loading, or moving a zero into that register. Another way to zero a register is to XOR it with itself. For example: XOR %eax, %eax. In x86_64 assembly, the XOR operation is faster than a MOV operation. Additionally, this ensures the register goes to zero. Otherwise, if you accidentally write to a memory cell containing the source value, then the result could be a non-zero value. I suppose this could be up for debate, since you could have code that inadvertently corrupts the XOR instruction itself. At any rate, the reason for doing this is speed, and a reduced probability of ending up with a non-zero value in your register.
Broken Code
Always remember the processor will do exactly what you tell it to do. Let’s say that you have a section of code that is not working. You cannot figure out why. Isolate the section of code that contains the problem, and look at it closely. If you still cannot figure out the problem, chances are that you have a conceptual error with one of the instructions. Look up each instruction in the problem area in the processor’s instruction set reference manual.
Make sure you understand the operation of each instruction. If you still cannot fix your code, then create a new program, and copy your problem area into this new program. That way, you can experiment with the code in a “sandbox” without corrupting your main project. If you still can’t figure it out, have someone else look at your code. Sometimes, I can look at a section of code a hundred times, and still not see the problem until someone else points it out. Then it’s obvious, and I can’t believe I didn’t catch it.
For more information, visit the Vintage Computer Category Page!
— Ricky Bryce