Introduction to the 1802 Membership Card Timer
In this section, we’ll crate a scan based 1802 Membership Card Timer using BASIC3. The 1802 Membership card uses the COSMAC 1802 Processor. Since we are basing the timer on the scan rate, you may have to vary the delay loops to calibrate your timer. In this case, I’m using the version which has a 4MHz resonator. Other versions have a potentiometer to adjust the clock frequency. In that case, I would recommend setting the pot as high as possible. Still, you may need to remove some delays if the clock is running too slow.
In this case, we’ll just count the number of seconds, and display this value to the LED’s. We’ll do this in BCD (Binary Coded Decimal). That way, it’s easier to decode the binary value. Additionally, if you have the 7 segment displays, they will display the correct number of seconds (0 to 99). Turn on switch 0 on the Membership Card to hold the value at 0. Shut off Switch 0 to start the timer.
Boot to BASIC3
Instead of Assembly, I’ll be doing this in BASIC3 this time. I have the MS20ANSJ ROM inserted, and running at 8000H. To boot to the ROM, simply enter “C0 80 00” at memory cells 0000, 0001, and 0002. Reset and run your membership card. When the menu appears, choose “B” (capitol) to enter the BASIC3 ROM. I’m starting with a cold boot (Capitol C). When you are finished loading the program, remember to type “RUN” in all capitol letters.
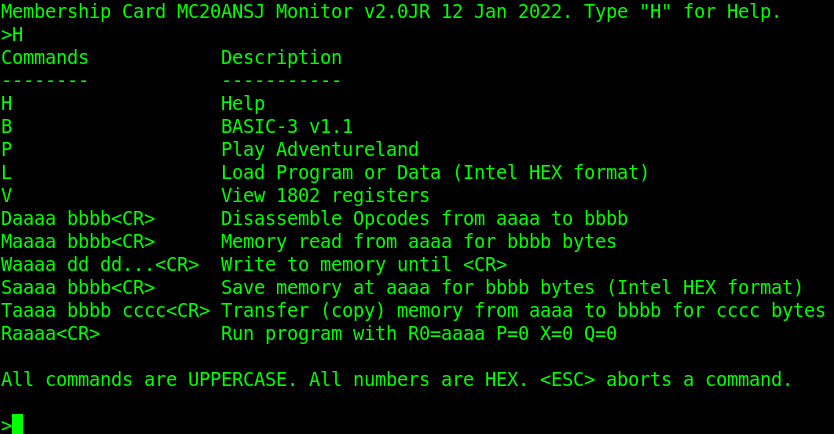
Enter the Code for the 1802 Membership Card Timer
At this point, we are ready to paste the code. Don’t forget to set the delays in your terminal for pasting code. I’m running the character and linefeeds both at 250ms. This will be slow, but reliable. In the future, you can experiment with lower delays. If you start getting errors, then you can increase the delay to the minimum that is reliable.
10 REM TIMER FOR COSMAC ELF MC (RICKY BRYCE)
20 LET A=0 : REM INITIALIZE A TO ZERO
30 FOR X=1 TO 29 : REM CREATE A DELAY LOOP
40 I=INP(0,4) : REM CHECK SWITCHES
50 IF I=1 THEN A=-1 : REM RESET COUNTER
60 NEXT X
70 C=C:C=C : REM DELAYS (FINE TUNE)
80 C=C:C=C:C=C:C=C
90 C=C:C=C:C=C:C=C
100 LET A=A+1 : REM INC A
110 GOSUB 150 : REM CHECK FOR HEX
120 OUT (0,4,A) : REM SEND OUTPUTS
130 IF A = 100 THEN A = -1 : REM RESET COUNTER
140 GOTO 30 : REM RESTART
150 REM HEX TO DECIMAL
160 B=A : REM BACKUP COUNTER
170 A=A AND 15 : REM REMOVE UPPER BITS
180 IF A=10 THEN GOTO 210 : REM INCREMENT 10'S
190 A=B : REM RESTORE A FROM BACKUP
200 GOTO 240 : REM EXIT SUBROUTINE
210 A=B : REM RESTORE A
220 A=A+16 : REM INCREMENT 10'S (HEX TO DECIMAL)
230 A=A AND 240 : REM KEEP ONLY UPPER BITS
240 RETURN : REM EXIT SUBROUTINE
Basically, in line 30-60, we are setting up a delay loop. This is one way to calibrate the timer. If your code runs too slow, then you can decrease the maximum value of X in line 30. Likewise, if the timer runs too fast, you can increase this time. Linex 70 – 90 are basically NO OPs. This is to finely calibrate the timer by causing more delay outside of the loop.
Line 100 is where we are incrementing A, which is our number of seconds. Since we don’t want the timer to display hex characters, I’ve set up a subroutine to handle this at line 150.
In line 120, we send the value of “A” to port 4. This is the LED’s and 7 segment display. After that we check to see if A is too high to display. If so, we reset it back to zero.
In lines 150 to 240, I’m not really converting a hex number to decimal. Instead, I’m just skipping over the extra hex characters. Basically, we’re just looking at the lower 4 bits, and once these hit the value of 10, we know to take more action.
If the lower 4 bits have the bit pattern for a 10, we simply add 16 to the timer. In Hex, this increments the “ten’s” place. After that, we just set the last 4 bits to zero.
If you would like to know how to do this in Assembly, you can check out this post as a guideline to get you started.
For other information, check out the COSMAC Category Page!
— Ricky Bryce