Introduction to Compiling Assembly Programs under CP/M for the Z80
In this post, we’ll discuss Compiling Assembly Programs under CP/M using Z80ASM. For this section, I’ll be using the CPUVille Single Board Computer.
Once you understand how to assemble a program, you can start writing your own programs. A good way to learn assembly is to start off with a very simple program. At this point, you can start learning more instructions, and improving your project. Before you know it, you will be familiar with the entire instruction set.
It’s important to realize that although the Z80 is compatible (for the most part) with the 8080, the instructions have different mnemonics. For example, a Move in the 8080 is now a Load in the Z80. This is due to a copyright on the 8080 instruction set.
In this example, we’ll write a simple “Hello World!” program.
Write the Code
We’ll start off by creating a label, CPM that points to 0005H. This will be the location of CP/M that we need to access to print our text to the screen. Write this in kwrite, or notepad., or any other text editor. You can even write this using Wordstar on the Z80 machine as a non-document file.
At this point, we’ll set the ORG at 0100H. This is the starting point for our program. All of your programs within CP/M will start at this address.
After that, we need to load a function code, 09H into the C register. This will tell the BDOS code that we want to output a string. You can find a list of the BDOS function codes for CP/M at this link.
Our next line, LD DE,TEXT loads the address of our TEXT label to the DE Register.
Our registers are all set up now, and we will CALL CPM to print our text to the display. This will end with the $, which is our termination character.
At last, we will return to CP/M.
CPM: EQU 0005H ; Address of BDOS
ORG 0100H ; This is where your CP/M Programs Start
LD C,09H ; This is the function code to print a string in BDOS
LD DE,TEXT ; This is the address of where our text begins
CALL CPM ; BDOS address (0005H)
RET ;RETURN TO CPM
TEXT: DEFB 48H, 45H, 4CH, 4CH, 4FH, 20H ;Hello
DEFB 57H, 4FH, 52H, 4CH, 44H, 21H ;World!
DEFB 0DH, 0AH, 24H ; Text terminates with 24H ($)
END
I’ll save this file as “helloworld.z80”.
Transfer Your File to the Z80 Computer
If you wrote this in Wordstar, you can skip this step, because the file is already on your Z80 Computer. If you wrote this in Linux or Windows, though, we will transfer the file to the computer using xmodem. We’ll do this with the PCGET command.

If you use minicom, you can use CTRL-A, then Z. In the menu press “S” to send via XModem. On the other hand, if you are using ExtraPutty, simply click “File Transfer” in the menu bar at the top of your screen. Browse for your file.
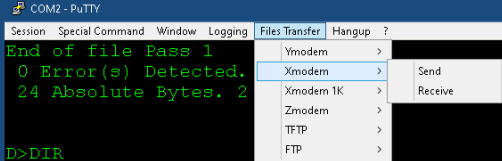
After the file transfer finishes, type “DIR” in CP/M to verify your file transfer.
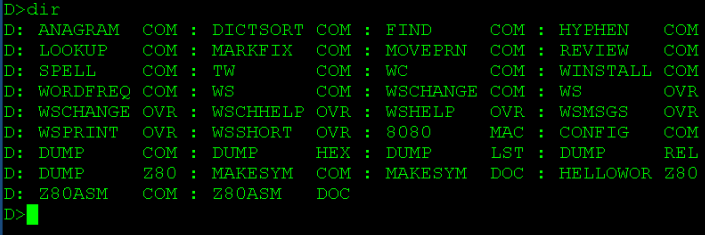
You will notice the file name is truncated. The actual file name on your machine is HELLOWOR.Z80. This is due to the name length restrictions in CP/M.
Compiling Assembly Programs under CP/M (HELLOWOR.Z80)
At this point, we are ready to run the z80asm compiler. This will create the .COM file that we can run. z80asm creates this automatically, but with some of the other compilers, you may have to run the “LOAD” command afterwards. In this case, our command is very simple. At the command prompt, on your Z80 machine, simply type “z80asm hellowor”. Type this without quotes and without the .z80 file extension.
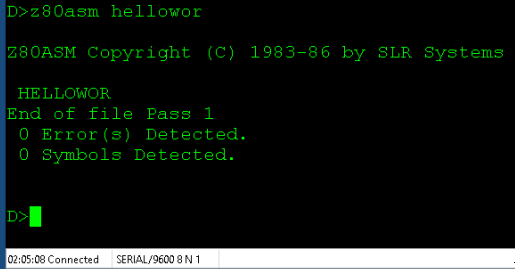
Now, type DIR, and you will see that z80asm created your .com file!
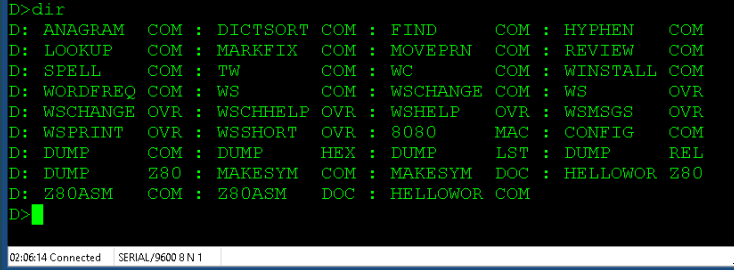
Run Your Program
To run your program, simply type, “hellowor” at your command prompt!
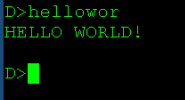
Summary for Compiling Assembly Programs under CP/M
In short, just be sure your program starts at 0100 with the ORG statement. After that, be sure you know the location of z80asm, and run the assembler passing the file name after the command. Pass the file name without the .Z80 extension. If you don’t have any errors, then you are ready to run your program! For a detailed description of each instruction, read the Z80 Manual.
For more information, visit the Vintage Computer Catagory Page!
— Ricky Bryce