Introduction to writing a Simple CP/M Console Program
Writing a Simple CP/M Console Program will get you started with Z80 Assembly under CP/M. We’ll just put together a simple program that asks what your name is, then print that name back to your terminal. The purpose of this exercise is to demonstrate how to write an assembly program to access the BDOS of CP/M. Additionally, you’ll learn how to compile a program using the Z80ASM Assembler under CP/M, and how to run this program. This will demonstration the Function 9 (write string) and Function 10 (read string) operations of BDOS.
Once you get started with a simple program like this, you can build on your code, and begin to learn the other Z80 processor instructions. The key point is that we just need to get started. After that, learning is very easy.
Writing the Program in Assembly
In this case, we’ll do all of the work on the Z80 machine. I’m using the Single Board Computer Kit from CPUVille. The same will work on an Altair 8800 or IMSAI 8080 with a Z80 processor. I’ll be using WordStar 4.0. You can use WordStar 3, as long as you apply the appropriate patches for your terminal. Within WordStar, we just need to create a non-document file. If you don’t have WorStar, you can just use a standard text editor on your local computer, then send the file to your Z80 machine. The compiler we’ll use expects the assembly code to end with the .z80 extension.
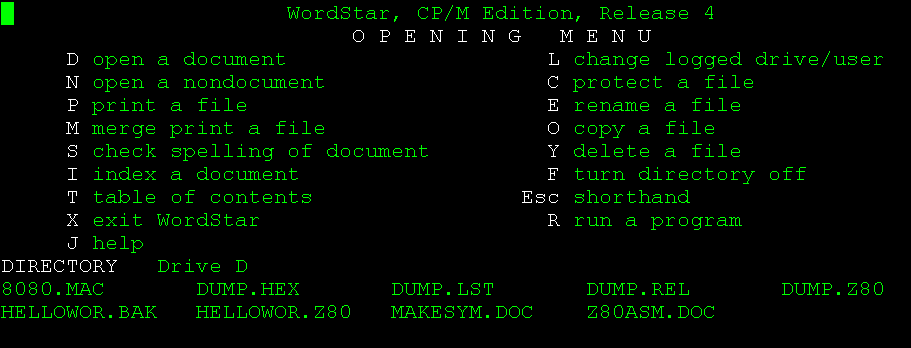
We’ll hit “N” within WordStar to start a non-document file.
We’ll just name this “TESTPROG.Z80”. WordStar will prompt you to create a new file.
TESTPROG.Z80 will contain the following code:
; Simple Program to ask your name and print this name to the console
BDOS: EQU 0005H
INBUFLEN EQU 100
CR: EQU 13
LF: EQU 10
ORG 0100H ; ALL CP/M PROGRAMS BEGIN HERE
; ASK THE USER HIS NAME
LD DE,ASK ; ASK USER WHAT HIS NAME IS
LD C,9 ; FUNCTION CODE TO WRITE STRING
CALL BDOS ; CALL BDOS TO WRITE TO CONSOLE
; GET THE USER'S NAME
LD DE,INBUF ; LOAD DE WITH ADDRESS OF CONSOLE INPUT BUFFER
LD A,INBUFLEN ; SET MAXIMUM STRING LENGTH
LD (DE), A ; STORE ACCUMULATOR TO BUFFER (MAX AT FIRST POSITION)
LD C, 10 ; FUNCTION CODE TO READ A STRING
CALL BDOS ; CALL BDOS TO READ FROM CONSOLE
; WRITE HELLO TO THE CONSOLE
LD DE, HANSWER ;
LD C,9 ; FUNCTION CODE TO WRITE STRING
CALL BDOS ; CALL BDOS TO PRINT STRING
; WRITE THE USER'S NAME TO THE CONSOLE
LD HL,INBUF+1 ; SET HL TO THE ADDRESS OF CHARS READ
LD D,0 ; UPPER DE PAIR TO 0
LD E, (HL) ; E CONTAINS THE NUMBER OF CHARACTERS READ
ADD HL,DE ; HL = HL + DE (DOUBLE ADD) GO TO THE END OF THE STRING
INC HL ; SET FOR ONE CHARACTER AFTER USER INPUT
LD A,'$' ; ADD TERMINATION CHARACTER TO END OF USER INPUT
LD (HL),A ; STORE TERMINATION CHARACTER AT END OF STRING
LD DE,INBUF+2 ; DE WILL BE THE ADDRESS OF THE FIRST CHARACTER
LD C,9 ; FUNCTION CODE TO PRINT STRING UNTIL TERMINATION
CALL BDOS ; CALL BDOS TO PRINT THE STRING TO THE SCREEN
RET ; RETURN TO CPM
ASK: DEFB 'WHAT IS YOUR NAME? $' ; DEFINE DATA BLOCK *
HANSWER: DEFB 13,10,'HELLO $' ; DEFINE DATA BLOCK *
INBUF: DEFS INBUFLEN+2 ; DEFINE DATA STRING *
END
When you are finished, simply hit CTRL-K in Wordstar, then X to save and exit.
Compile the Simple CP/M Console Program
At this point, we are ready to compile the Z80 program. I’m using the Z80ASM from this link. This Z80 Assembler will run under CP/M. Since the assembler is on the same drive as my testprog.z80 file, I’ll simply type “z80asm testprog” without the quotes.
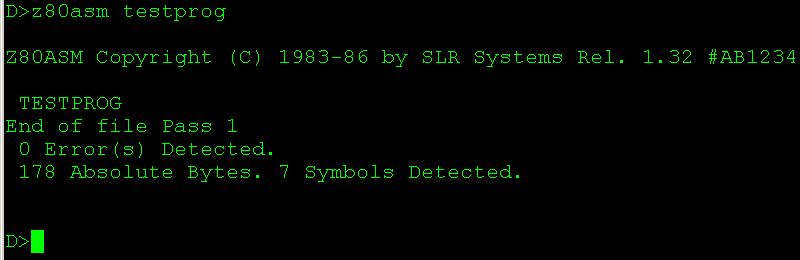
As you can see, we don’t have any errors.
Running the Program
Let’s check our directory listing to ensure that we have a testprog.com file. Z80asm creates this file.
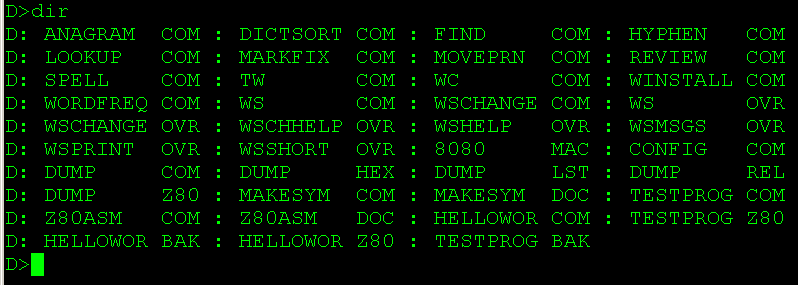
Finally, to run the file, simply type “testprog”.
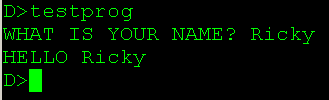
Now that you understand how the basic console functions work, you can write assembly code to read user input, and to display data to the user!
For more information, visit the Vintage Computers Category Page!
— Ricky Bryce