Introduction to Calculating PID in BASIC
In this section, we’ll be Calculating PID in BASIC to find the values for Proportional, Integral, and Derivative. BASIC is a very common programming language that has been around since the 70’s. This code should run on most computers with basic installed. Particularly, I’m running Microsoft BASIC (MBASIC).
We’ll just use Allen Bradley’s rule of thumb when making our calculation. This will give us a good starting point. Obviously every process is a little different, but I’ve found this method to work well for most processes. If you choose to use a purely mathematical method, try the Ziegler-Nichols method instead.
Keep in mind that by using this method, we require the loop to be unstable for a short period of time. Basically we just zero out the integral and derivative. After that, we’ll keep bumping up the proportional gain until the loop becomes unstable. The loop is unstable when it has continuous oscillations, or if the oscillations are getting worse. We call this a “Heuristic Method”… Basically that means that we need to do some guessing to find the right value for Proportional.
It’s important to realize that in order to tune a PID, we need to cause disturbances to see how the loop reacts whenever we make a change.
Keep in mind, that these procedures are just rules of thumb. Use a more precise tuning method when there is danger of damage to machinery or people!
PID Tuning Procedure
As I said before, we’ll have to do some guessing at first. We’re doing this to find the right value for Proportional. First, start bumping up the proportional gain. Be sure to cause a disturbance each time we bump the proportional gain. You can do this by a small change in the setpoint, or by changing the load on the system. You will want to find the lowest value of proportional gain that causes the loop to become unstable. We call this the critical value (Kcrit).
At this point, you will want to record the natural period. This is the amount of time it takes to complete one oscillation. You can measure the amount of time peak to peak. Similarly, you can measure trough to trough as well. We’ll use this value to find a starting point for both Integral, and Derivative if we use it.
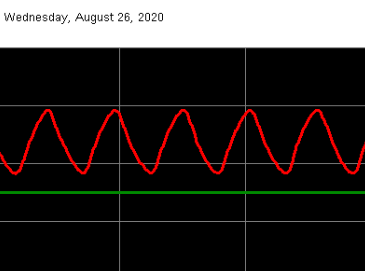
Dependent Mode
For Dependent mode, set Kc to half the value of Kcrit. Additionally, the natural period you recorded earlier will be for Ti (Integral). One eighth of the natural period will be the setting for derivative (Td). Keep in mind that very few processes even use derivative. You don’t want it whenever you have a noisy process variable. For example, a flow control system. When you set Ti, and Td, be sure the time units mach (ie: minutes or seconds).
Independent Mode
The values for Independent mode require some extra calculations. We set Kp (proportional gain) the same way. In other words, it’s still one half of Kcrit. The variable is a little different for Integral, which is Ki. Set Ki using the following formula: Ki=Kp/Natural Period. If you choose to use derivative, I’ll usually use this formula: Kd = Kp * (Natural Period/8). Once again, just be sure the time units match up.
Write the Program
I’m writing this program in Microsoft Basic, and I’m using it on my IMSAI 8080. The program asks a few questions, then should give you a good starting point for your variables.
10 REM PID CALCULATION PROGRAM
20 INPUT "What is the value of Kcrit"; KCR
30 INPUT "What is the natural period in seconds"; NP
40 INPUT "Are you in Dependent or Independent mode (d/i)";MODE$
50 INPUT "Are your controller units in Minutes or Seconds (m/s)";TBASE$
60 IF TBASE$ = "m" THEN NP=NP/60
70 IF MODE$="I" THEN GOTO 120
80 PRINT "Set the value of Kc to " KCR/2
90 PRINT "Set the value of Ti to ";NP
100 PRINT "Set the value of Td to "; NP/8
110 END
120 LET KP = KCR/2
130 PRINT "Set the value of Kp to ";KP/2
140 PRINT "Set the value of Ki to ";KP/NP
150 PRINT "Set the value of Kd to ";KP*(NP/8)
160 END
Ok
If you find any errors in the code, please let me know.
Summary of Calculating PID in BASIC
In short, keep safety first. Write down your old PID settings before you try anything. Also be sure there is no risk of damage to equipment, product or people when using this method. In this case, I always remove integral and derivative. Run Proportional gain up until the loop becomes unstable. Record the natural period, and make note of the time units and formula that your PID is using. In other words, the formula can be dependent (standard), or independent (traditional).
For more information, visit the ControlLogix Category Page!
— Ricky Bryce